Design Algorithm Analysis GATE CS and IT previous year questions with Answer
Ques 1 Gate 2024 Set-2
The chromatic number of a graph is the minimum number of colours used in a proper colouring of the graph. The chromatic number of the following graph is _________
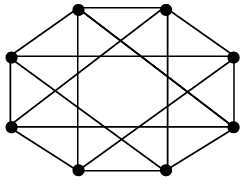
a is the correct answer.
Ques 2 Gate 2024 Set-2
The number of distinct minimum-weight spanning trees of the following graph is _________
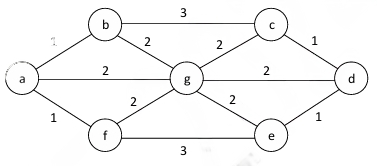
a is the correct answer.
Ques 3 Gate 2022
Consider the following recurrence:
f(2n) = 2f(n) -1, for n≥1;
f(2n+1) = 2f(n) +1, for n≥1;
Then, which of the following statements is/are TRUE?
Ques 4 Gate 2022
Which one of the following statements is TRUE for all positive functions f (n) ?
Ques 5 GATE 2021 SET-1
Let P be an array containing n integers. Let t be the lowest upper bound on the number of comparisons of the array elements, required to find the minimum and maximum values in an arbitrary array of n elements. Which one of the following choices is correct?
Ques 6 GATE 2021 SET-1
Consider the following three functions.
f1 = 10n
f2 = nlogn
f3 = n√n
Which one of the following options arranges the functions in the increasing order of asymptotic growth rate?
Ques 7 Gate 2020
What is the worst case time complexity of inserting n elements into an empty linked list, if the linked list needs to be maintained in sorted order ?
Ques 8 Gate 2020
What is the worst case time complexity of inserting n2 elements into an AVL-tree with n elements initially ?
Ques 9 Gate 2020
For parameters a and b, both of which are ω(1), T(n)=T(n1/a)+1, and T(b)=1. Then T(n) is
Ques 10 Gate 2020
The number of permutations of the characters in LILAC so that no character appears in its original position, if the two L’s are indistinguishable, is ________ .
12 is the correct answer.