C Programming GATE CS and IT previous year questions with Answer
Ques 1 Gate 2024 Set-1
Consider the following C program:
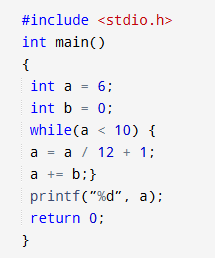
Ques 2 Gate 2024 Set-1
Consider the following C program:
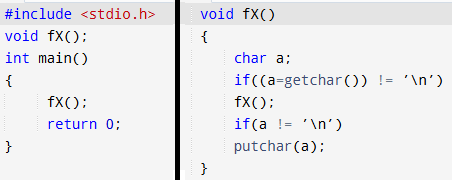
Ques 3 Gate 2022
What is printed by the following ANSI C program?
int main(int argc, char *argv[])
{
int a[3][3][3] =
{{1, 2, 3, 4, 5, 6, 7, 8, 9},
{10, 11, 12, 13, 14, 15, 16, 17, 18},
{19, 20, 21, 22, 23, 24, 25, 26, 27}};
int i = 0, j = 0, k = 0;
for( i = 0; i < 3; i++ ){
for(k = 0; k < 3; k++)
printf(“%d”, a[i][j][k]);
printf (“\n”);
}
Ques 4 Gate 2022
What is printed by the following ANSI C program?
int main(int argc, char *argv[])
{
int x = 1, z[2] = {10, 11};
int *p = NULL;
p = &x;
*p = 10;
p = &z[1];
*(&z[0] + 1) += 3;
printf("%d, %d, %d ", x, z[0], z[1]);
return 0;
}
Ques 5 Gate 2020
Consider the following C functions.
int i;
for (i = 0; b>0; i++) {
if (b%2)
arr [i] = 1;
else
arr[i] = 0;
b = b/2;
}
return (i);
}
int pp(int a, int b) {
int arr[20];
int i, tot = 1, ex, len;
ex = a;
len = tob(b, arr);
for (i=0; i
tot = tot * ex;
ex= ex*ex;
}
return (tot) ;
}
The value returned by pp(3,4) is ________ .
81 is the correct answer.
Ques 6 Gate 2020
Consider the following C functions.
{
static int i= 0;
if (n > 0) {
++i;
fun1(n-1);
}
return (i);
}
int fun2(int n) {
static int i= 0;
if (n>0) {
i = i+ fun1 (n) ;
fun2(n-1) ;
}
return (i);
}
55 is the correct answer.
Ques 7 Gate 2020
Consider the following C program.
#include <stdio.h>
int main () {
int a[4][5] = {{1, 2, 3, 4, 5},
{6, 7, 8, 9, 10},
{11, 12, 13, 14, 15},
{16, 17, 18, 19, 20}};
printf("%dn", *(*(a+**a+2)+3));
return(0);
}
The output of the program is _______
19 is the correct answer.
Ques 8 Gate 2019
Consider the following C program:
int main() {
int a[] = {2, 4, 6, 8, 10};
int i, sum = 0, *b = a + 4;
for (i = 0; i < 5; i++ )
sum = sum + (*b - i) - *(b - i);
printf("%d\n", sum);
return 0;
}
10 is the correct answer.
Ques 9 Gate 2019
Consider the following C program:
int main() {
float sum = 0.0, j = 1.0, i = 2.0;
while (i / j > 0.0625) {
j = j + j;
printf("%f ", sum);
};
return 0;
}
The number of times variable sum will be printed When the above program is executed is _________ .
a is the correct answer.
Ques 10 Gate 2019
Consider the following C program:
int main(){
int arr[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 0, 1, 2, 5}, *ip = arr + 4;
printf("%dn", ip[1]);
return 0;
}
The number that will be displayed on execution of the program is _________ .
a is the correct answer.